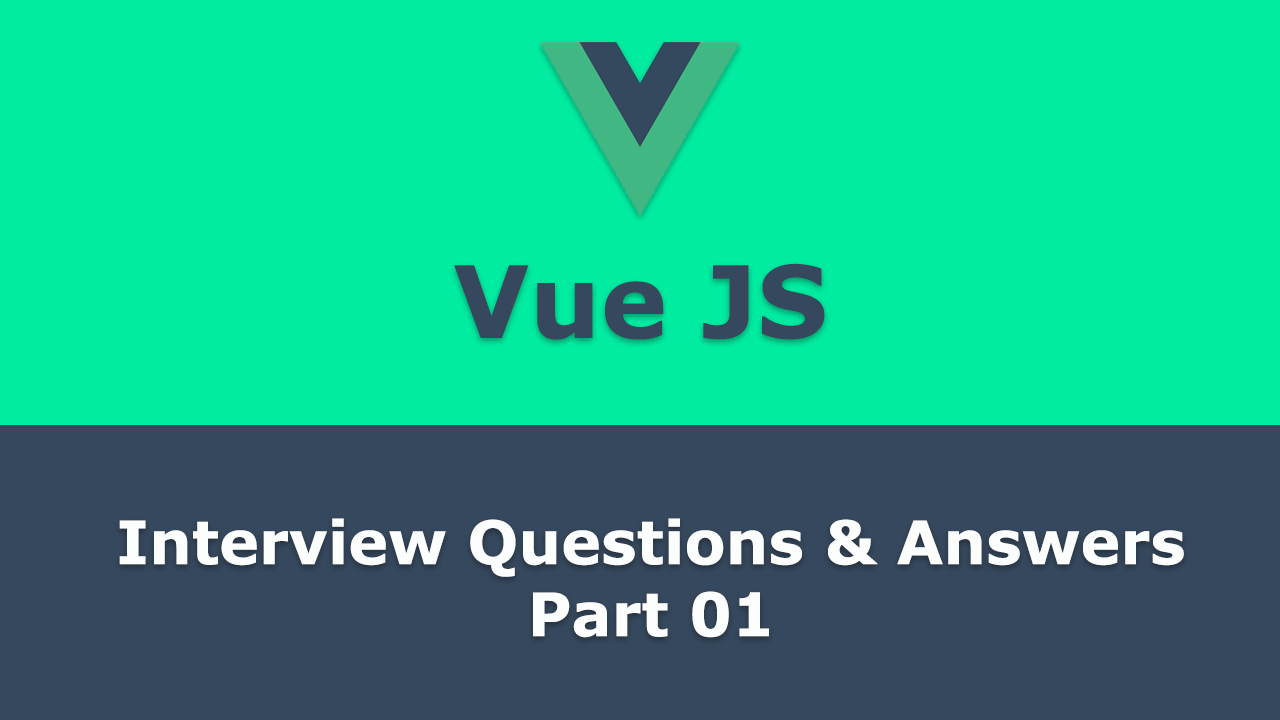
Frequently asked - Vue JS Interview Questions and Answers - Part 01
1. What is Vue.js? What are the advantages of it?
Vue is a progressive framework used to building user interfaces.The core library is focused on the view layer only, and is easy to pick up and integrate with other libraries or existing projects.
Following are the advantages of using Vue.js.
- Small in size - The size of this framework is 18 to 21KB and it takes no time for the user to download and use it.
- Easy to Understand - One of the reasons for the popularity of this framework is that it is quite easy to understand. The user can easily add Vue.js to his web project because of its simple structure.
- Simple Integration - Vue.js can be integrated with the existing applications easily.
- Flexibility - This flexibility also makes it easy to understand for the developers of React.js, Angular.js, and any other new JavaScript framework.
- Virtual DOM - It uses virtual DOM similar to other existing frameworks such as ReactJS, Ember etc. Virtual DOM is a light-weight in-memory tree representation of the original HTML DOM and updated without affecting the original DOM.
- Components - Used to create reusable custom elements in VueJS applications.
- Two-Way Communication - Vue.js also facilitates two way communications because of its MVVM architecture which makes it quite easy to handle HTML blocks.
2. What is Vue instance and how to create it?
Every Vue application starts by creating a new Vue instance with the Vue
function:
var vm = new Vue({
// options
})
As a convention, we often use the variable vm
(short for ViewModel) to refer to our Vue instance. When we create a Vue instance, we pass in an options object. A Vue application consists of a root Vue instance created with new Vue
, optionally organized into a tree of nested, reusable components.
3. What is data in Vue instance?
When a Vue instance is created, it adds all the properties found in its data object to Vue’s reactivity system. When the values of those properties change, the view will “react”, updating to match the new values. It is recommended to initialize all the data properties that needs to be reactive upfront in the data
option.
// Our data object
var data = { a: 1 }
// The object is added to a Vue instance
var vm = new Vue({
data: data
})
4. What are all the life cycle hooks in Vue instance?
Each Vue instance goes through series of steps when they are created, mounted and destroyed. Along the way, it will also runs functions called life cycle hooks, giving us the opportunity to add our own code at specific stage. Below are the events, a Vue instance goes through.
-
beforeCreate - The first hook in the creation hooks. They allow us to perform actions before our component has even been added to the DOM. We do not have access to the DOM inside of this.
-
created - This hook can be used to run code after an instance is created. We can access the reactive
data
. But templates and Virtual DOM have not yet been mounted or rendered. -
beforeMount - The beforeMount hook runs right before the initial render happens and after the template or render functions have been compiled. Most likely we’ll never need to use this hook.
-
mounted - We will have full access to the reactive component, templates, and rendered DOM. This is the most frequently used hook.
-
beforeUpdate - This hook runs after data changes on our component and the update cycle begins. But runs right before the DOM is patched and re-rendered.
-
updated - This hook runs after data changes on our component and the DOM re-renders. If we need to access the DOM after a property change, here is probably the safest place to do it.
-
beforeDestroy - This hook will run right before tearing down the instance. If we need to clean up events or reactive subscriptions, this is the right place to do it.
-
destroyed - This hook will be used to do any last minute clean up.
5. How do you bind the data in Vue.js?
We can bind the data using “Mustache” (i.e. {}
) syntax which is the basic form of data binding in Vue.js.
<h1>{{ title }}</h1>
The mustache content will be replaced with the value of the title
property on the corresponding data object. It will also be updated whenever the data object’s title
property changes.
6. How do you do one-time binding?
To bind a data only once, we can use v-once
directive. This will also affect all the other bindings in the same node.
<h1 v-once>{{ title }}</h1>
7. How do you do two-way data binding?
We can use the v-model
directive to create two-way data bindings on form input, textarea, and select elements. It automatically picks the correct way to update the element based on the input type.
<input v-model="message" placeholder="edit me">
<p>Message is: {{ message }}</p>
v-model
will ignore the initial value, checked or selected attributes found on any form elements. It will always treat the Vue instance data as the source of truth.
8. What is directives in Vue.js?
A directive is some special token in the markup that tells the library to do something to a DOM element. It is same like Angular directives but simpler than that. Directives are prefixed with v-
and are expected to be a single JavaScript expression.
<p v-if="seen">Now you see me</p>
Here, the v-if
directive would remove/insert the <p>
element based on the truthiness of the value of the expression seen
.
9. What are arguments and modifiers in directives?
Some directives can take an “argument”, denoted by a colon after the directive name. For example, the v-bind
directive is used to reactively update an HTML attribute:
<a v-bind:href="url"> ... </a>
Here href
is the argument, which tells the v-bind
directive to bind the elements href
attribute to the value of the expression url
.
Another example is the v-on
directive, which listens to DOM events:
<a v-on:click="doSomething"> ... </a>
Here the argument is the event name to listen to. Modifiers are special postfixes denoted by a dot, which indicate that a directive should be bound in some special way. For example, the .prevent modifier tells the v-on
directive to call event.preventDefault()
on the triggered event:
<form v-on:submit.prevent="onSubmit"> ... </form>
10. How do you create a custom directive in Vue.js?
In addition to the default set of directives like v-model
and v-bind
, we can also create and register our own directives. The syntax for registering a directive is as follow:
// Register a global custom directive called `v-focus`
Vue.directive('name', {
// When the bound element is inserted into the DOM...
inserted: function (el) {
// Focus the element
el.focus()
}
})
Then in a template, we can use the newly created directive v-name
as attribute on any element, like this:
<div v-name></div>
11. What are all the directive hook functions?
A directive definition object can provide below optional hook functions.
-
bind: It is called only once, when the directive is first bound to the element. This is where we can do one-time setup work.
-
inserted: This is called when the bound element has been inserted into its parent node.
-
update: It is called after the containing component’s VNode has updated, but possibly before its children have updated. The directive’s value may or may not have changed, but we can skip unnecessary updates by comparing the binding’s current and old values.
-
componentUpdated: It is called after the containing component’s VNode and the VNodes of its children have updated.
-
unbind: This is called only once, when the directive is unbound from the element.
All these directive hooks are passed with four arguments namely: el
, binding
, vnode
, and oldVnode
.
12. Explain the directive hook arguments in details.
Directive hooks are passed with these below arguments:
el: The element the directive is bound to. This can be used to directly manipulate the DOM.
binding: An object containing the following properties.
-
name: The name of the directive, without the
v-
prefix. -
value: The value that is passed to the directive.
-
oldValue: The previous value of the directive, but only available in
update
andcomponentUpdated
-
expression: The expression of the binding as a string.
-
arg: The argument passed to the directive, if any. For example in
v-my-directive:foo
, the arg would befoo
. -
modifiers: An object containing modifiers, if any. For example in
v-my-directive.foo.bar
, the modifiers object would be{ foo: true, bar: true }
. -
vnode: The virtual node produced by Vue’s compiler.
-
oldVnode: The previous virtual node, only available in the
update
andcomponentUpdated hooks
.
For simple directives where we want the same behavior in update
and bind
, we can simply pass a function instead of directive definition object.
Vue.directive('color-swatch', function (el, binding) {
el.style.backgroundColor = binding.value
})
13. What are the conditional directives?
Vue.js provides set of directives to manipulate the DOM such as add/remove, show/hide. The below are the available directives:
v-if
: it adds or removes the element from the DOM based on the given expression. For example:
<button v-if="isLoggedIn">Logout</button>
v-else
: it is similar like else
in the native if
condition, which will render the DOM element only if the adjacent v-if
resolves into false
. We don’t need to pass any value to this.
<button v-if="isLoggedIn">Logout</button>
<button v-else>Login</button>
v-else-f
: This directive is used when we need more than two options to be checked. For example, ifLoginDisabled
property is disabled then we need to prevent user to login instead just display the label. This can be achieved through v-else
statement.
<button v-if="isLoggedIn">Logout</button>
<button v-else="isLoginDisabled">Login disabled</button>
<button v-else>Login</button>
v-show
: This directive is similar to v-if
but it renders all elements to the DOM and then uses the CSS display property to show/hide elements instead of completely add/remove the DOM. This directive is recommended if the elements are switched on and off frequently.
<span if-show="user.name">Welcome user, {{user.name}}</span>
14. What is the difference between v-show and v-if directives?
Below are some of the main differences between between v-show
and v-if
directives:
-
v-if
only renders the element to the DOM if the expression passes whereasv-show
renders all elements to the DOM and then uses the CSS display property to show/hide elements based on expression. -
v-if
supportsv-else
andv-else-if
directives whereasv-show
doesn’t support else directives. -
v-if
has higher toggle costs since it add or remove the DOM everytime whilev-show
has higher initial render costs. i.e,v-show
has a performance advantage if the elements are switched on and off frequently, while thev-if
has the advantage when it comes to initial render time. -
v-if
supports tab butv-show
doesn’t support.
15. What is the purpose of v-for directive?
This directive allows us to loop through the items of an array or object. Example of an array usage:
<ul id="list">
<li v-for="(item, index) in items">
{{ index }} - {{ item.message }}
</li>
</ul>
var vm = new Vue({
el: '#list',
data: {
items: [
{ message: 'John' },
{ message: 'Locke' }
]
}
})
Example of an object usage:
<div id="object" v-for="(value, key, index) in object">
{{ index }}. {{ key }}: {{ value }}
</div>
var vm = new Vue({
el: '#object',
data: {
user: {
firstName: 'John',
lastName: 'Locke',
age: 30
}
}
})
16. What is key
in Vue.js?
In order to render DOM elements more efficiently, Vue.js reuses the elements instead of creating them from scratch every time. This default mode is efficient, but in some cases it may causes problems. For example, if you try to render the same input element in both v-if
and v-else
blocks then it holds the previous value as below:
<template v-if="loginType === 'Admin'">
<label>Admin</label>
<input placeholder="Enter your ID" key="admin-id">
</template>
<template v-else>
<label>Guest</label>
<input placeholder="Enter your name" key="user-name">
</template>
17. Why should not use if and for directives together on the same element?
It is recommended not to use v-if
on the same element as v-for
. Because v-for
directive has a higher priority than v-if
. There are two common cases where this can be tempting:
-
To filter items in a list (e.g.
v-for="user in users" v-if="user.isActive"
). In these cases, replaceusers
with a new computed property that returns your filtered list (e.g.activeUsers
). -
To avoid rendering a list if it should be hidden (e.g.
v-for="user in users" v-if="shouldShowUsers"
). In these cases, move the v-if to a container element (e.g.ul
,ol
).
18. How do you pass an object to a directive?
We can pass in a JavaScript object literal to a directive since directives can take any valid JavaScript expression.
<div v-demo="{ color: 'white', text: 'hello!' }"></div>
Vue.directive('demo', function (el, binding) {
console.log(binding.value.color) // => "white"
console.log(binding.value.text) // => "hello!"
})
19. What is computed property?
In Vue.js, a computed property is a getter function of the property. Conside the following example:
var vm = new Vue({
el: '#example',
data: {
message: 'Hello'
},
computed: {
// a computed getter
greetMessage: function () {
// `this` points to the vm instance
return this.message + ', Welcome.'
}
}
})
In the template:
<div id="example">
<p>Original message: "{{ message }}"</p> <!-- Hello -->
<p>Greet message: "{{ greetMessage }}"</p> <!-- Hello, Welcome. -->
</div>
Computed properties are by default getter-only, but we can also provide a setter when we need it.
20. What is the difference between computed properties and methods?
Computed properties are getter function in Vue instance rather than actual methods. we can define the same function as a method instead. However, the difference is that computed properties are cached based on their dependencies. A computed property will only re-evaluate when some of its dependencies have changed. In comparison, a method invocation will always run the function whenever a re-render happens.
When we have to compute something by doing lot of computations like looping through a large array, it is best to use computed properties instead of a method. Without caching, we would spend more time than necessary. When we do not want cache, we can use a method instead.