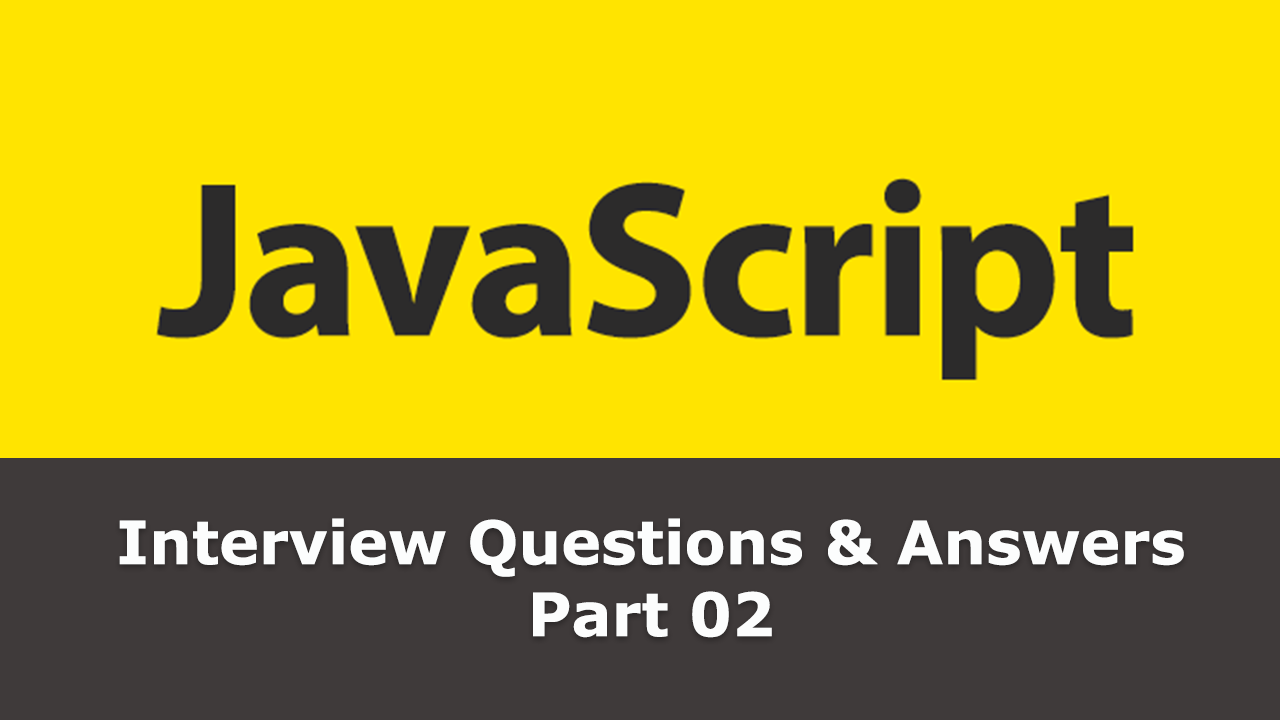
Frequently asked - JavaScript Interview Questions and Answers - Part 02
21. What is the difference between window.onload
and onDocumentReady
?
onDocumentReady
event is fired when DOM is loaded and it is safe to do any DOM manipulation after this event, so that the element is in the DOM. It will run prior to image and external contents are loaded.
window.onload
will wait until the entire page is loaded including its CSS, images and fonts.
22. What is exception handling?
Exception handling is handling run time error in program. JavaScript provides try...catch
statement to mark a block of code that can throw error in run time. If an exception is thrown at try
block, the control will shifts to catch
block. If no exception thrown, the catch block will be skipped.
There is also one additional block, finally
, at the end. It will run regardless of whether the try
block failed or not.
23. What is shift()
and push
? How they are differing from each other?
The shift()
removes the first element from an array and return it. On the otherhand, unshift()
adds the given element at the start of an array.
The push()
adds the given element at the end of the array. And, pop()
removes the last element from an array.
24. What is arguments
object in JavaScript?
The arguments
object is a local object available by default within all functions. This object contains the list of all the arguments that are passed to the function. And we can access them with an index value from 0, like arguments[0]
.
The arguments object is not an Array. It is similar to an Array, but does not have any Array properties except length. To convert them into array, we can do:
var args = Array.from(arguments);
var args = [...arguments];
25. What is callback? How they work?
JavaScript allows a regular function to be passed as an argument to another function. This callback function will be invoked later inside the passed function. It is also called high-order functions. A common usecase for callback is event handlers.
$("#btn").click(clickEvent);
How they work: We are not passing the function with ()
. Because we are passing only the function definition. So that it will be invoked later. Since callback functions are closures. They have access to the containing function’s scope, and even from the global scope.
26. What is typeof
operator?
It is an unary operator, means it will have only one operand. It will return the data type of the operand, like “string” “number” or “boolean”. The resulting type is always string.
Bonus: The typeof null
return object even though it is not an object. From the first version of the JavaScript, the typeof
checks the operand’s type tag which is 1-3 bits (like, 000 for object, 1 for int and 100 for string) stored with values. null
was the machine code NULL pointer or an object type tag, so it returns object.
27. What is Browser Object Model or BOM?
This is used to access the Browser window. Using the global object window
, we can access the browser. They provide many useful methods like:
window.console
: accessing the console of the browserwindow.alert
,window.confirm
andwindow.prompt
: showing alerts or getting input from the user.window.history
,window.navigator
: accessing history API and getting browser informations.window.location
: redirecting user or change urls.
28. What is negative infinity?
The negative infinity is a number in JavaScript, which can derived by dividing a negative number by 0.
Negative infinity is less than zero when compared, and positive infinity is greater than zero.
29. What is new
operator in JavaScript?
The new
operator is used to construct a user-defined object from a function. The this
inside the function is set to the new object. So any assignments we are not with the this
will be added as a property to this object.
function Car(name, model)
this.name = name; //this refers here the object that is created now.
this.model = model;
}
var myCar = new Car('Audi', 'a3');
console.log( myCar.name ) //Audi
If we explicitly return something inside the function, then the this
binding will be ignored and an empty object will be assigned to the caller variable.
30. What is this
keyword in JavaScript? Explain in details.
By simply saying, this
refers to the object that is bind to the function at the runtime. To understand which object will be bound to the function, we should consider below situations:
Default binding: This happens when a function is invoked in stand alone.
num = 5;
function printNumber()
console.log( this.num );
}
When invoking the printNumber()
, the system prints 5. Because, here this
resolves to the global object window
. So window.num
yields 5 here.
Implicit binding: In this case, when the function has a context-object, this
resolves to the owning object or containing object. The below example illustrates this.
num = 5;
function printNumber()
console.log( this.num );
}
var obj =
num: 6,
printNumber: printNumber
};
When we call the object obj
’s printNumber method, obj.printNumber()
, we will get 6
. Because the obj
is the this
for the printNumber()
.
Explicit binding: By this way we are forcing a function to use an object to resolve the this
. This can be achieved by using call
and apply
.
function printNumber()
console.log( this.num );
}
var obj =
num: 6
};
printNumber.call( obj ); //6
In the above code, we are invoking the printNumber
function with call and explicitly binding the obj
object to it. So the result will be 6
.
The methods call
and apply
are identical, but they behave differently with their parameters. apply
expects the parameters to be in array format, where call
expects separately.
new
binding: The new
binding is invoked when the function is invoked with new
keyword. We are using new
keyword in JavaScript to construct an object out of a function.
function Person(name)
this.name = name
}
var person1 = new Person('foo');
console.log( person1.name ); // foo
When we call a function with new
keyword, a new object is constructed and set this newly constructed object to this
in the function.
Final Note: this
is referring the object to be bound for an executing function, but determining the object is based on from where and how the function was called. Other than this, ES6 arrow functions have lexical scope, in which they refer the enclosing function. Additionally, in event handlers the this
is set to the element which fired the event.
31. When is JavaScript synchronous? And when is asynchronous?
JavaScript is always synchronous and single-threaded. If we are executing a block of code in JavaScript, then no other code will run at the given same time.
JavaScript is only asynchronous in the sense that it can make, for example, Ajax calls. In that case, JavaScript make the Ajax request and will run with other code and does not block the execution for return value. Upon the call returns a value, JS will run the callback and no other code will run at this point.
JavaScript’s setTimeout
and setInterval
also operates with this same kind of callback. So it’s more accurate to say that JavaScript is synchronous and single-threaded with various callback mechanisms.
jQuery Ajax has an options to make the calls synchronous. But this is problematics and allows to more traditional programming.
32. What is bind
in JavaScript? How it is used?
bind
is used to bind an object to the function at run time and force it to use the passed object for this
when invoked.
function printName()
console.log( this.name );
}
var obj = name: 'Javascript' };
printName.bind(obj)(); //Javascript, or
var b = printName.bind(obj);
b(); //Javascript
bind()
is helpful in cases where you want to create a shortcut to a function which requires a specific this
value.
33. What is constructor
in JavaScript?
This refers to the object constructor function that created the instance object.
All objects will have a constructor property. Objects created without the explicit use of a constructor function, will have the constructor
property that points to the fundamental object constructor. Following is an example of an object created without the constructor function.
var o = };
o.constructor === Object; // true
When object created with constructor function, constructor
will points to the function.
function Car(name)
this.name = name;
}
var mycar = new Car('Honda');
mycar.constructor === Car; //true
34. What is instanceof
in JavaScript?
This operator tests whether an object has the prototype property of a given constructor in its prototype chain. For example,
function Car(name)
this.name = name;
}
var mycar = new Car('Honda');
mycar instanceof Car; //true
mycar instanceof Car;
returns true
since the mycar is constructed from the Car
.
35. What is Number
and String
Objects in JavaScript?
JavaScript have Number
and String
objects to work with the primitive types number and string. We don’t need to explicitly create and use Number
and String
objects. JavaScript uses them to convert the primitive types to object when we are accessing any properties or methods on the primitive types. For example,
var str = 'Javascript';
str.length //10
Here, we are declaring a variable str
and assign a primitive string to that. When accessing the length property, the primitive types actually does not have the length
property, but JavaScript compiler convert this primitive string to Object string, and the return the length
property of it. To say more clearly, JavaScript returns new String(str).length
. And this String object will be removed immediately from the memory once it returns the required value. So we won’t see any difference. Remember, declaring a string as var str = 'Javascript';
is different from declaring as String object as var str = new String('Javascript');
. The latter will return object.
var str = new String('Javascript');
typeof str; //object
36. How can you implement additional methods or functions to the core Object in JavaScript? How do you extend core objects in JavaScript?
In JavaScript, objects can have properties dynamically added to them. To add a property or method to an object, we should add them to the prototype property of the object. Consider the below example,
String.prototype.titleCase = function()
return this.split(' ').map(function(elm)
return elm[0].toUpperCase() + elm.slice(1, elm.length);
}).join(' ');
Now we can use titleCase
method for all the strings, like 'javascript programming'.titleCase();
results Javascript Programming
.
37. What is asynchronous programming, and why is it important in JavaScript?
In Synchronous programming, the code is executed sequentially, line by line and it is blocking other code and wait for a function to complete.
Asynchronous programming in JavaScript, which is opposite to Synchronous, means the code will be run in the event loop. When a operations which can block the execution is needed such as a server call, it will start the function and keeps running the other code without blocking for the result. When the response is ready, an interrupt is fired and an event handler will run.
This is important in JavaScript, because it is a very natural fit for user interface code, and very beneficial to performance on the server.
38. What is encodeURI
and decodeURI
in JavaScript?
The encodeURI
is used to encode an URL by replacing special characters, like space, percent. For example,
encodeURI('www.example.com/this is a test url with %character')
will results in, www.example.com/this%20is%20a%20test%20url%20with%20%25character
. Notice that the space and percentage symbol are replaced with %20
and %25
. The decodeURI
works in reverse way.
39. What is createDocumentFragment()
? How to use that?
DocumentFragments
are DOM Nodes. But they are never part of the main DOM tree. The usual use case is to create the document fragment, append elements to the document fragment and then append the document fragment to the DOM tree.
Consider, if we are going to create hundred list elements and append them into the DOM, adding them one by one in DOM by a for
loop, is much expensive. Instead, we can create an DocumentFragments
and add the list items into them. Then finally, we append the fragment elements to the actual DOM. This way we largely reduced the DOM manipulation and increased the performance. The example code is,
var element = document.getElementById('ul'); // assuming ul is exists
var fragment = document.createDocumentFragment();// creating a fragment
var technologies = ['HTML', 'CSS', 'Javascript', 'jQuery', 'Angular'];
technologies.forEach(function(technology)
var li = document.createElement('li');li.textContent = technology;
fragment.appendChild(li);//adding to the fragment not the actual DOM
});
element.appendChild(fragment); //Now appending the list to the DOM
40. What are all the best practices for writing JavaScript Code? How do you write better code?
This has many different answers. Some of the best ways are:
- Always initialize the variables before you use them.
- Always use
===
equals instead of==
. - Wrapping code that could thrown errors at run time and feature-based code in
try...catch
block. - Don’t pollute global scope and don’t use global variables.
- Always use
"use strict";
. - Use lint tools to validate JavaScript code and avoid any potential risks.
- Wrap your code in an anonymous function or Immediately Invoked Function Expressions (IIFE), so that it will affect the global scope.
- Give clear name for variables and functions.
- Give comments wherever needed so that the other developer understands the code better.
- Place all your scripts at end.
- Reduce DOM manipulations and if you need to add large dom elements by script, use
document.createDocumentFragment
.