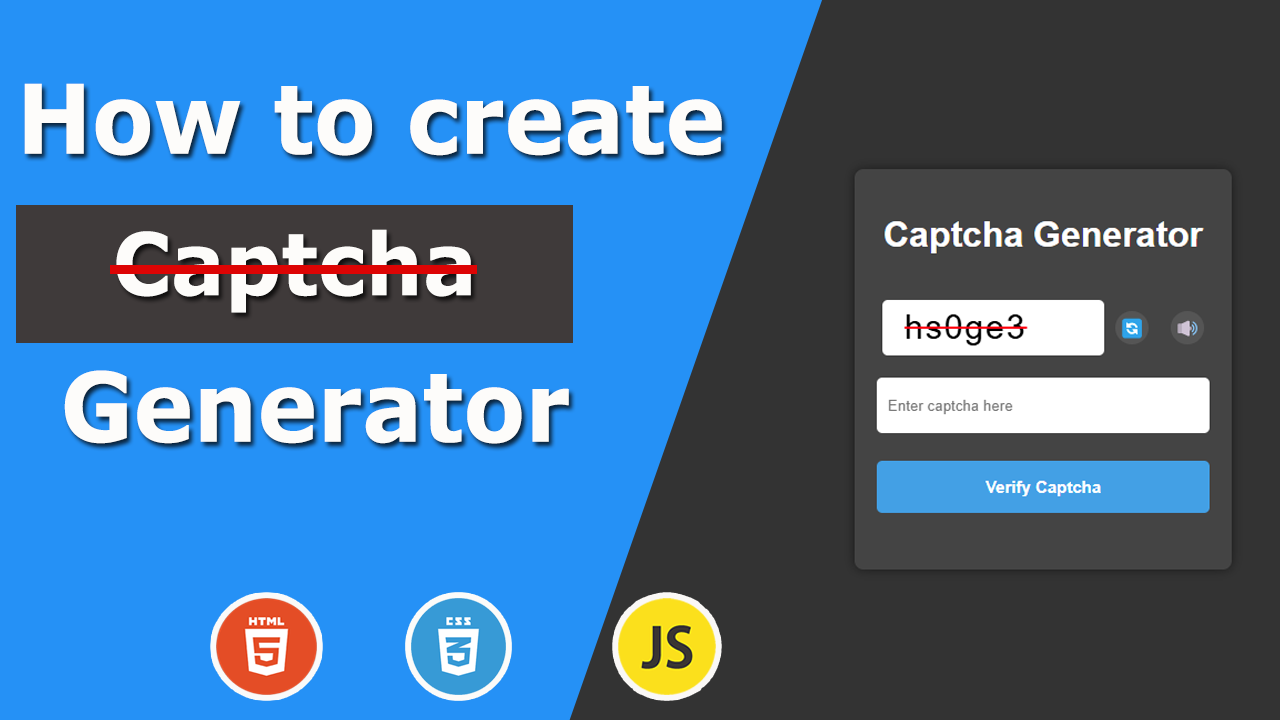
December 16, 2024 • 4 min read
Building a CAPTCHA Generator with JavaScript, HTML, and CSS
In this tutorial, we'll create a CAPTCHA generator tool using JavaScript, HTML, and CSS. The tool will feature a user-friendly UI and provide functionality to generate and verify CAPTCHAs. This can be a fun project for beginners and a useful addition to secure web forms.
Features of the CAPTCHA Tool:
- User Interface: A modern card with a title, CAPTCHA display, input field, and buttons styled with CSS.
- Captcha Features:
- Display CAPTCHA as text with a strike-through effect.
- Buttons to refresh and read CAPTCHA aloud.
- Verification System: Validate user input and display success or error messages.
Step 1: Setting Up the HTML Structure
The HTML structure contains a container for the CAPTCHA, an input field, and buttons for user interaction.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Captcha Generator</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="captcha-container">
<h1>Captcha Generator</h1>
<div class="captcha-display">
<canvas id="captchaCanvas" width="200" height="50"></canvas>
<div class="captcha-actions">
<button id="refreshCaptcha" aria-label="Generate New Captcha">🔄</button>
<button id="readCaptcha" aria-label="Read Captcha">🔊</button>
</div>
</div>
<input type="text" id="captchaInput" placeholder="Enter captcha here">
<button id="verifyCaptcha">Verify Captcha</button>
<p id="captchaMessage"></p>
</div>
<script src="script.js"></script>
</body>
</html>
Explanation:
<canvas>
: Displays the CAPTCHA text with a strike-through effect.- Buttons: ”🔄” for generating a new CAPTCHA and ”🔊” for reading the CAPTCHA aloud.
- Input and Verification: Input field for entering CAPTCHA and a button to validate.
Step 2: Styling with CSS
The CSS ensures a responsive, modern UI with clear visual feedback.
body {
margin: 0;
font-family: Arial, sans-serif;
background-color: #333;
color: #fff;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
h1 {
margin-bottom: 2.5rem;
}
.captcha-container {
text-align: center;
background-color: #444;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.5);
width: 300px;
}
.captcha-display {
display: flex;
align-items: center;
justify-content: center;
margin-bottom: 20px;
border-radius: 15px;
}
canvas {
background-color: #fff;
text-decoration: line-through;
font-size: 20px;
letter-spacing: 2px;
}
input[type="text"] {
width: calc(100% - 20px);
padding: 10px;
margin-bottom: 10px;
border: none;
border-radius: 5px;
}
button {
padding: 10px 15px;
background-color: #555;
color: #fff;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #666;
}
#captchaMessage {
margin-top: 10px;
}
.captcha-actions {
display: inline-flex;
align-items: center;
gap: 10px;
}
.captcha-actions button {
display: flex;
align-items: center;
justify-content: center;
margin-left: 10px;
width: 30px;
height: 30px;
background-color: #555;
border-radius: 50%;
border: none;
cursor: pointer;
color: #fff;
font-size: 18px;
}
.captcha-actions button:hover {
background-color: #666;
}
#captchaCanvas {
border-radius: 5px;
}
#captchaInput {
height: 30px;
}
#verifyCaptcha {
width: 100%;
padding: 15px;
margin: 15px 0;
background-color: #2fa3e9;
color: #fff;
font-size: 15px;
font-weight: bold;
border: none;
border-radius: 5px;
cursor: pointer;
}
#verifyCaptcha:hover {
background-color: #2491d4;
}
Key Features:
- Centered Card Design: The
.captcha-container
is centered with padding and shadow for aesthetics. - CAPTCHA Area:
<canvas>
styled with a white background and a strike-through line. - Buttons and Input: Full-width, responsive, and visually distinct for easy interaction.
Step 3: Adding Functionality with JavaScript
The JavaScript handles CAPTCHA generation, rendering, validation, and reading aloud.
const canvas = document.getElementById("captchaCanvas");
const ctx = canvas.getContext("2d");
const refreshCaptcha = document.getElementById("refreshCaptcha");
const readCaptcha = document.getElementById("readCaptcha")
const captchaInput = document.getElementById("captchaInput")
const verifyCaptcha = document.getElementById("verifyCaptcha");
const capthcaMessage = document.getElementById("captchaMessage");
let generatedCaptcha = "";
function generateCaptcha() {
generatedCaptcha = Math.random().toString(36).substring(2, 8);
renderCaptcha();
}
function renderCaptcha() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.font = "30px Arial";
ctx.fillStyle = "#000";
ctx.fillText(generatedCaptcha, 20, 35);
const textWidth = ctx.measureText(generatedCaptcha).width;
ctx.strokeStyle = "#f00";
ctx.lineWidth = 2;
ctx.beginPath();
ctx.moveTo(20, 25);
ctx.lineTo(20 + textWidth, 25);
ctx.stroke();
}
verifyCaptcha.addEventListener("click", () => {
const userInput = captchaInput.value.trim();
if (userInput === generatedCaptcha) {
captchaMessage.textContent = "You have entered the correct captcha!";
captchaMessage.style.color = "#00ff00";
} else {
captchaMessage.textContent = "Incorrect captcha. Try again.";
captchaMessage.style.color = "#ff0000";
}
});
refreshCaptcha.addEventListener("click", generateCaptcha);
readCaptcha.addEventListener("click", () => {
const utterance = new SpeechSynthesisUtterance(generatedCaptcha);
speechSynthesis.speak(utterance);
});
generateCaptcha();
Key Functionalities:
- Generate CAPTCHA:
- A random alphanumeric string is generated.
- Rendered on a canvas with a strike-through line.
- Refresh and Read CAPTCHA:
- Refresh button generates a new CAPTCHA.
- Read button uses the SpeechSynthesis API to read aloud the CAPTCHA.
- Verification:
- Compares user input with the generated CAPTCHA.
- Displays success or error messages.
Conclusion
This CAPTCHA generator is a great addition to your web projects and a fun way to learn HTML, CSS, and JavaScript. Try customizing it further for advanced use cases like integrating it into a form submission process.