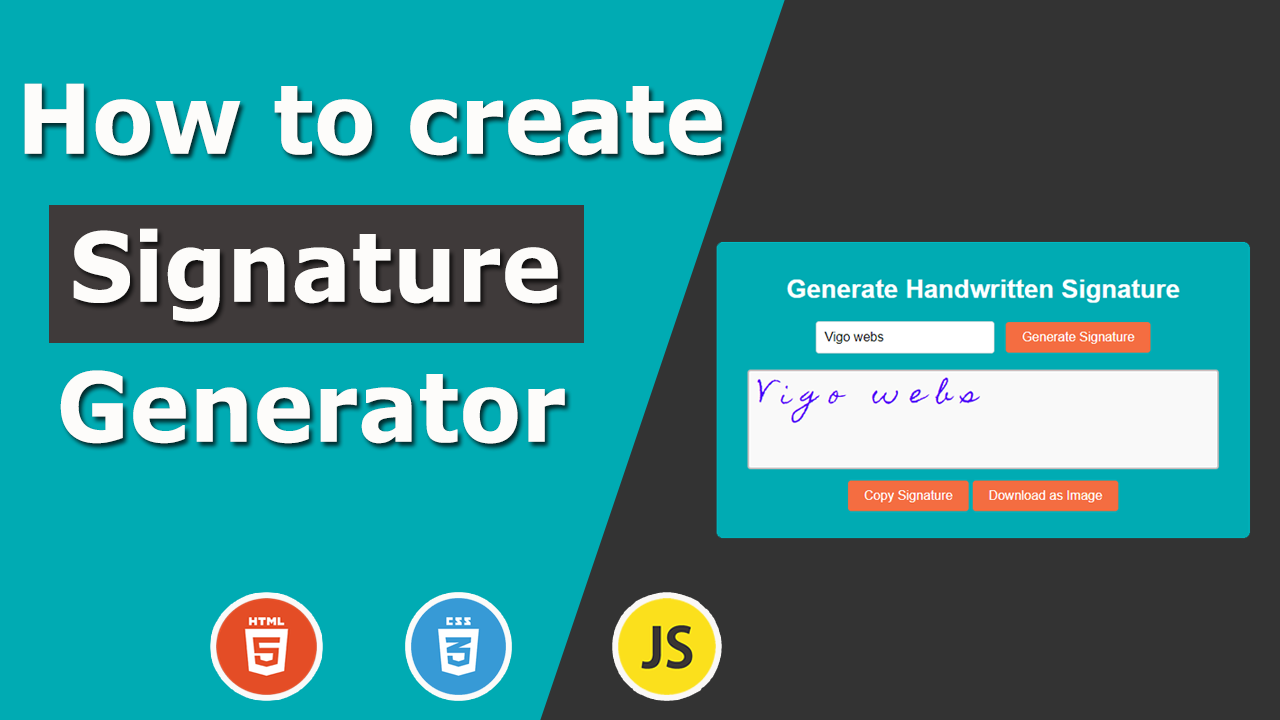
Creating a Handwritten Signature Generator with Downloadable Image using HTML, CSS, and JavaScript
In this tutorial, we will create a simple web application where users can input their name, generate a handwritten-style signature, and download it as an image. This is a fun and useful tool that can be implemented easily using HTML, CSS, and JavaScript.
We’ll cover:
- Setting up the HTML structure for name input and buttons.
- Styling the form and signature using CSS.
- Adding JavaScript to generate and display the signature.
- Implementing a feature to download the signature as an image.
Let’s dive in!
1. Setting Up the HTML Structure
Our form will include:
- An input field for the user to enter their name.
- A button to generate the signature.
- A textarea to display the signature.
- A button to copy the signature to the clipboard.
- A second button to download the signature as an image.
Here’s the basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Name to Signature</title>
<link rel="stylesheet" href="styles.css">
<link href="https://fonts.googleapis.com/css?family=Homemade+Apple" rel="stylesheet">
</head>
<body>
<div class="container">
<h1>Generate Handwritten Signature</h1>
<div class="input-section">
<input type="text" id="nameInput" placeholder="Enter your name" />
<button id="generateButton">Generate Signature</button>
</div>
<textarea id="signatureOutput" readonly placeholder="Your signature will appear here"></textarea>
<button id="copyButton">Copy Signature</button>
<button id="downloadButton">Download as Image</button>
<p id="copyMessage"></p>
<!-- Hidden canvas element to generate the signature image -->
<canvas id="signatureCanvas" style="display:none;"></canvas>
</div>
<script src="script.js"></script>
</body>
</html>
This structure sets up the form and defines all the elements we need. We’ll style and add functionality to these elements next.
2. Styling the Form and Signature with CSS
Now, let’s add some basic styles to make the form look appealing. The signature output will be displayed in a cursive font to resemble a handwritten signature.
body {
font-family: Arial, sans-serif;
background-color: #333;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.container {
text-align: center;
background-color: #00abb4;
padding: 20px;
border-radius: 8px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
}
h1 {
color:#fff;
}
.input-section {
margin-bottom: 20px;
}
#nameInput {
padding: 10px;
font-size: 16px;
width: 200px;
border-radius: 4px;
border: 1px solid #ddd;
margin-right: 10px;
}
#generateButton {
background-color: #f46d38;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
}
#generateButton:hover {
background-color: #ef7e50;
}
#signatureOutput {
width: 90%;
height: 100px;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ddd;
border-radius: 4px;
font-family: 'Homemade Apple', cursive; /* Custom handwritten font */
font-size: 32px;
color: blue;
line-height: 1.4;
letter-spacing: 1px;
background-color: #f9f9f9;
border: 2px solid #bbb;
resize: none;
overflow: hidden;
}
#copyButton, #downloadButton {
background-color: #f46d38;
color: #fff;
padding: 10px 20px;
border: none;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
}
#copyButton:hover, #downloadButton:hover {
background-color: #ef7e50;
}
#copyMessage {
color: #fff;
margin-top: 10px;
font-size: 14px;
}
Here we:
- Styled the input, buttons, and the textarea that displays the signature.
- Applied a cursive font (Homemade Apple from Google Fonts) to the signature textarea, making it look handwritten.
3. Adding JavaScript to Generate the Signature
Now that we have the structure and style in place, let’s add JavaScript to:
- Generate the signature when the user clicks the “Generate Signature” button.
- Copy the signature to the clipboard when clicking the “Copy Signature” button.
Here’s the JavaScript:
// Generate signature and display it in the textarea
document.getElementById('generateButton').addEventListener('click', function() {
const name = document.getElementById('nameInput').value.trim();
const signature = name ? name.split('').join(' ') : 'Enter a valid name!';
document.getElementById('signatureOutput').value = signature;
drawSignatureToCanvas(signature); // Draw to canvas when generating
});
// Copy signature to clipboard
document.getElementById('copyButton').addEventListener('click', function() {
const signatureText = document.getElementById('signatureOutput');
signatureText.select();
document.execCommand('copy');
document.getElementById('copyMessage').textContent = 'Signature copied!';
});
This script generates the signature by appending a space between each letter of the name and displays it in the textarea. Additionally, the copy function lets users copy the signature to their clipboard with one click.
4. Download the Signature as an Image
Finally, we’ll add the ability to download the signature as an image (PNG format). We will use an HTML <canvas>
element to draw the signature and convert it into an image.
Add this additional script to draw the signature on the canvas and enable the download:
// Download signature as an image
document.getElementById('downloadButton').addEventListener('click', function() {
const canvas = document.getElementById('signatureCanvas');
const link = document.createElement('a');
link.download = 'signature.png'; // File name for download
link.href = canvas.toDataURL('image/png'); // Create image URL from canvas
link.click(); // Trigger download
});
// Draw the signature text onto the canvas
function drawSignatureToCanvas(signature) {
const canvas = document.getElementById('signatureCanvas');
const ctx = canvas.getContext('2d');
const canvasWidth = 400;
const canvasHeight = 100;
canvas.width = canvasWidth;
canvas.height = canvasHeight;
// Clear the canvas before drawing
ctx.clearRect(0, 0, canvasWidth, canvasHeight);
// Set canvas text style (matching the signature style)
ctx.font = "32px 'Homemade Apple', cursive";
ctx.fillStyle = "blue";
ctx.textAlign = "center";
ctx.textBaseline = "middle";
// Draw the signature text onto the canvas
ctx.fillText(signature, canvasWidth / 2, canvasHeight / 2);
}
Explanation:
-
Canvas: A hidden canvas (
#signatureCanvas
) is used to draw the signature text in a style that matches the textarea display. -
Download as Image: When the “Download as Image” button is clicked, the canvas content is converted into a PNG image, and the download is triggered automatically.
Conclusion
By following these steps, you have created a fully functional name to handwritten signature generator with the ability to download the signature as an image! This tool is useful for anyone who wants a quick, stylish signature for digital documents or fun projects.
Feel free to customize the design or add more features, such as saving different font styles or colors for the signature. With HTML, CSS, and JavaScript, the possibilities are endless.
Let me know if you need further assistance or additional customization!